Organic Reaction Simulator
It is a tool that simulates the organic reactions and automatically generate the IUPAC names for the organic compounds drawn by you. This covers most of the syllabus of the Organic Chemistry for G.C.E. A/L examination.
Features
- Nice Canvas to draw your Organic compounds.
- Generates IUPAC names for the drawn component as possible. (It generates IUPAC names for almost all the compounds which G.C.E A/L examination expect students to know)
- You can simulate reaction for organic compounds with preferred inorganic/organic compounds and the selected conditions. (Currently supporting 168 reactions)
- Extensibility of adding IUPAC naming rules without the need of recompiling the code.
- Extensibility of adding custom reactions, again without compiling a single line of code.
Here is a screen shot of the main panel when ‘Aldole reaction’ is simulated for some simple reactants.

Aldole Reaction - Reaction Simulator
The Drawing Panel
The ‘Reaction Simulator’ app provides 2 views for the user. One is the main panel which shows and manages reactions which is shown in the above figure. The other view is the drawing panel. It will be not much different from your favorite drawing application.

Drawing Panel - Reaction Simulator
Here you can select elements and bond types to draw your organic compound. Additionally It has tools to move or delete selected parts of your drawing.
The other thing you may have already noticed, you don’t need to complete all the bonds for a particular element. Rather if you keep one side of a single bond unconnected, it will be automatically connected to a ‘H'(Hydrogen) at the rendering, similarly for double bond the default connecting element would be ‘O'(Oxygen), and for triple bond it is ‘N’ (Nytrogen). And you don’t even need to bother about connecting bonds with an element, as if you connected only one bond to ‘C’ all the other 3 possible bonds will be considered as connected with ‘H’s.
Just check the drawing panel on your own and find its user friendliness. Now after you finish designing your compound, just press “RETURN”.

IUPAC Name Generator - Reaction Simulator
Yea it will nicely render our compound, and look at the bottom, it has generated the IUPAC name for the compound.
The Main Panel
This contain a canvas that you can add, edit and delete organic compounds (so you will be directed to the ‘Drawing Panel’), some sidebar panels to select Inorganic compounds and additional conditions requires for the reactions and a set of buttons to start reactions and manage the result set. At the end of the sidebar there is a ‘help’ button that directed you to the user guide.
Extending IUPAC Naming Rules
If you go to the “Data/IUPAC/” directory (you can view svn from here, http://svn.dimuthu.org/organic_chemistry/Organic_Reaction_Simulator/Data/IUPAC/) you can find there are set of .txt files (infact xmls) that defines the IUPAC rules.
If you open one of them (we will take al.txt), it would be something like this
<IUPAC basename="al" subname="oxo" level="6" nonumber="true" affectto="1">
<bond type="2">
<element type="7">
</element>
</bond>
<bond type="1">
<element type="1">
</element>
</bond>
</IUPAC>
It is the naming rules for aldehyde compounds. (H-C=O). First it identify the the aldehyde by checking double bond to ‘O’ (which is the element type ‘7’), and a single bond to ‘H’ (which is the element type ‘1’). If the element group found, it will give the name ‘al’ or ‘oxo’ depending on whether it defines the main group of elements of the compound or not.
If you take a look at all these rules, you can have a good idea what each of these syntax mean. And may be you can add your own rules, if you find something missing or incorrect there.
Extending the Reactions
The reaction rules are stored in the “Data/Reactions/” directory ( http://svn.dimuthu.org/organic_chemistry/Organic_Reaction_Simulator/Data/Reactions/).
I will take the first reaction we studied in the ‘Organic Chemistry’ Class.
CH4 + Cl2 + hv (dim light) —————-> CCl4 + H2
Here is the rule defining that reaction. (cl2hv.txt)
<reaction inorganics="Cl2" conditions="hv">
<check>
<bond type="1">
<element type="H">
</element>
</bond>
</check>
<reordering activity="remain">
<bond type="1">
<check>
<element type="H">
</element>
</check>
<reordering activity="remain">
<element type="H">
<reordering activity="replace" to="Cl">
</reordering>
</element>
</reordering>
</bond>
</reordering>
</reaction>
If you take a close look at, what it says is if there exist inorganic ‘Cl2’ and condition ‘hv’ (reactions inorganics=”Cl2″ conditions=”hv”), check for a ‘H’ (element type=”H”) elements that is connected to a ‘C’ element (which is the root of the XML) through a single bond (bond type=”1″), then don’t replace the ‘C’ (reordering activity=”remain”) and don’t replace the bond type (reordering activity=”remain”), just replace the ‘H’ with ‘Cl’ (reordering activity=”replace” to=”Cl”). It is so simple as that.
These rules are applied to all the C elements in reactants. Look at the following figure for this rule in application.
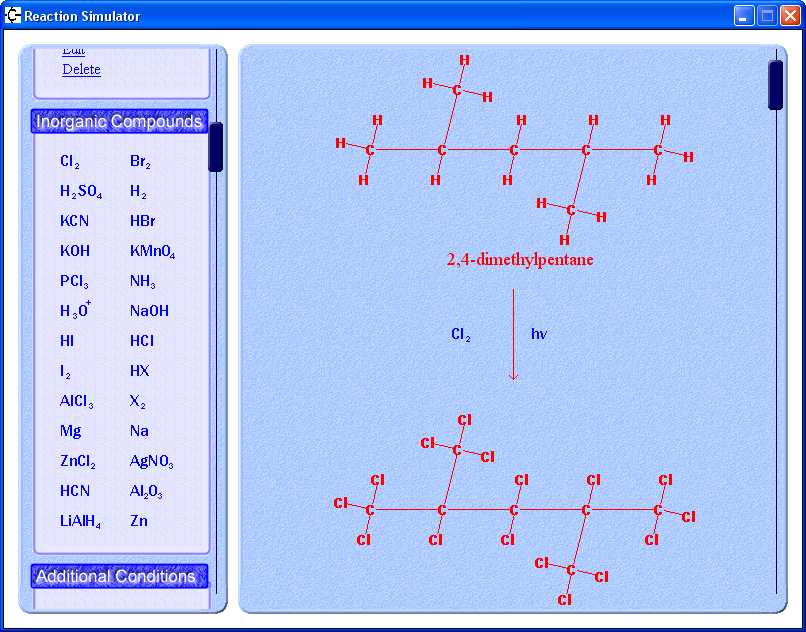
Alkane + Cl2 Reaction - Reaction Simulator
These reaction rules can be as complex as you want. Specially when two or more reactants are involved in a reaction, rules defining that reaction will be little complex. Look at how aldole reaction (which is applied in the first figure of this post) is written in aldole.txt.
There are all together 168 reactions currently defined in this way. If you found some reaction is missing, feel free to add a another rule file defining that reaction. And if you want to share that with others, just let me know :), so I can integrate it in to the distribution.
Download
This software application is not yet in a official release. I just thought use this post to do a pre release of the ‘Reaction Simulator’. You can download the windows binary of the pre release version (343KB) from http://downloads.dimuthu.org/bins/chemistry/reaction_simulator_pre_release/reaction_simulator_pre_release.zip
Source Code
SVN location for the source code of ‘Reaction Simulator’ is http://svn.dimuthu.org/organic_chemistry/Organic_Reaction_Simulator/
Known Limitations – Possible Improvements
- Generation of IUPAC names and simulations of reactions are not supported for compounds which involves Benzene ring.
- IUPAC names generation is not supported for cyclic compounds which are anyway not part of the G.C.E. A/L syllabus.
- The set of elements, available in drawing compounds, set of inorganic compounds and conditions, available in reactions are fixed and cannot be extended without changing the code and recompiling.
- You can’t start with an IUPAC name and derive the compound. Currently you always have to start with drawing the compound and then generate the IUPAC.
- Only for windows!
Little Background
4 years ago, When I was a level 2 student in the University of Moratuwa, I participated to a competition for making educational software tools organized by C.S.E and N.I.E (National Institute of Education). I submitted a software that teaches Organic Chemistry. It consisted of interactive tutorials targeting local G.C.E A/L exams with exercises and revisions in both Sinhala(My Mother tongue) and English languages(not in Unicode though). I got the second price for that.
After the award I decided to improve my software application by adding an ‘Organic Reaction Simulator’. In fact in the vacation of 2 weeks for the New Year, I could complete it.
Although the N.I.E supposed to distribute the applications submitted to the competition throughout the country, it didn’t happened. So I decided to publish at least the ‘Reaction Simulator’ application which I actually didn’t submitted to the competition.
Technologies Used
This is completely written in C++. It hasn’t use MFC, because I thought MFC is too heavy for such a small application. I used a lightweight, small image library code taken with some custom changes from some windows game programming book.
This is using XML to load data about reactions and IUPAC names which I have described in details in the early part of the blog. It uses a small inbuilt xml parser (just one recursive function) to parse these xmls.
So it has no depenedencies to third party libraries, You can just chekcout the souce code from the svn, open the visual studio project and compile it (press ‘F7’).